Jacob Baytelman
Building software since 1998
Innovations of Tomorrow Projects Contact meTechnical assessment of software developers
It is normal to believe that an exam can help to qualify skills.
It is poor to believe that theoretical multiple choice test can be a really valuable assessment means,
at least in software development.
I remember my university professor saying "the best practice is theory", which is probably true in some circumstances but for his wisdom we preferred to treat him as our "queer old dean" rather than "dear old queen".
We realise how amazingly someone plays piano when we enjoy the performance, not when we participate in discussions about solfeggio. We have high regards for doctors if they relieve our pains, not if they chew over correct treatment methods.
Multiple choice testssuck are particularly poor.
For example, if I am asked, which function in PHP returns the number of elements in an array: size, sizeof, length, I start hesitating. I simply do not remember. I have been coding in 10 different languages for several different platforms for more than 15 years already and I cannot keep in my memory all syntax in detail. Simply there's no need to do it, because:
- we have code auto completion (hints), so we can always choose from the provided popups.
- we, developers, (I am probably telling you now our biggest secret) work in "write once-copy many times" mode. We all have our own sets of libraries, codes, functions which we bring along to each new project and simply copy-paste code snippets.
-we have compilers and error reporters. When a mistake gets into the code (a wrong function or type or number of parameters), the code will not compile and you receive an error with a pointer to exact line where this error sits.
In a multiple choice test I have to choose, I cannot show my work process and cannot explain what I think.
Or another example, you are shown some code and asked to find an error in it or say what it will output. No problem if the code is quite simple but if we have an SQL script with several joins and cases in conditions, it gets really annoying. I have no compiler in my head. When I write an SQL query I do it step by step, build simple blocks, test them on the database, see the errors and fix them. Finally I have a bigger chunck of code, efficient in work but hard for human reading.
So I put there comments. Comments help me remember what this piece of code is about and if I come back to it in 3 years, I will know exactly what it is for and why it was constructed this very and not the opposite way. Or if someone else has to deal with my code, my comments provide the context in a human-friendly format. Have you ever seen multiple choice tests with in-code comments? I have not.
Even worth, tests through at you pieces of code with meaningless methods, variables and classes - no context at all - something like
B = A->foo(c);
If in the real life someone writes such code, he or she deserves been fired immediately.
Development skills should be tested on a real task, in a real working environment: computer + Internet (to be able to check the syntax if needed) + compiler or web server to see immediately how you code works. An alternative is to have a discussion so the tester can see how you react, think, approach problems.
Another good field is object oriented. Don't forget, it has been a holy war arena for ages and the sords are still sharp on both sides. I still remember the times when object-oriented was not so much in fashion (and somehow complex software solutions for space ships worked without object oriented implementations).
Some of my products were written in a procedural approach though the languages allowed object-oriental and they were delivered in time and on budget. Some “theory-savvy” guys laughed at me and my ignorance, but my products are still online while theirs failed initial tests and were rejected. Done is better than perfect.
Of course, candidates have to understand the principles of encapsulation, inheritance and polymorphism, but why on Earth should I test them for these if the project has no hierarchy of objects, everything is quite “flat”?
Now here are my questions for a PHP developer tests:
1. You have a string of values, separated by commas, how to convert it to an array?
I am happy to hear: there're explode and implode functions, I do not remember which is which, but there's php.net and I can check it in 5 secs.
2. Is PHP case sensitive?
I am happy to hear: yes and no. Variables are yes, functions are not, but to be on the safe side, I will code as if everything is case sensitive. The same for any other language. Just for the sake of the discipline.
3. What is an SQL injection and how to prevent it?
I am happy to hear: when through user input some malicious code is sent, it appears in the SQL query and is executed with it. In order to prevent it, we have to filter all user inputs on the server side (even if it's already validated on the client side), and for my personal paranoia, also each incoming parameter to each function must be also validated. And we should check the data types (cast if necessary), check for the reasonable length (and trim), filter out dangerous characters (slashes, quotes, etc). And we have to implement our own logs and log all incoming data and outgoing results (surely we need to have a switch to turn the logs on and off when needed).
4. What happens if 2 simultaneous processes try to update the same database record? And what to do with it?
I am happy to hear: depends on the database and its settings. The second one can wait (good - they both will work, bad - the execution time suffers). Or there might be a dead lock and data losses, uncommitted reads and execution time suffers again. Transactions or isolation levels can help. And all this is good to do in stored procedures.
5. What to do if you work with WordPress and some theme for it and you need to do customization or find a bug in this theme?
I am happy to hear: for customization we need to create a child out of this theme and do the changes in the child. If the original theme is updated, our changes will not suffer.
6. How to post a request from a php script to a remote server and get the result, assume, it's XML and then parse it?
I am happy to hear: there's CURL library, quite powerful, it supports POST and GET methods, caching and sessions and we can even simulate user login to a remote site and then parse the data we receive and in case of XML we have XML parser. I do not remember details, but I am not banned on php.net.
7. Imagine we have 2 web servers with our PHP application and they sit behind a load balancer. A user logins successfully and then in some point in time the user is refused in further actions with “you are not authorised” message. We checked all the system in the test environment, everything is ok. What to do?
I am happy to hear: probably in your test environment there's no load balancer. The problem is that the load balancer can send 1st request to the 1st server and the 2nd to the 2nd, so the 1st request is login and a session is created for this user on the 1st server, but the second request comes to the 2nd server where there's no their session. This happens because by default PHP stores sessions in files. A quick and simple fix is to modify the session management so that it would work with the database, after all the 2 web servers “speak” to the same database.
8. What is AJAX, please show me you implementation?
I am not happy: to see AJAX example based on jQuery and other libraries. It might be good at work, but I want to see native JS code.
I am happy to see something like this:
9. If you develop a widget for a web site an you need a specific version of jQuery, but there might be already loaded some other version of jQuery (by other widgets or by the site itself), so conflicts are possible. What to do?
I am happy to hear: wrap my code in its own namespace.
10. Tell me about your work environment.
I am happy to hear: I have a LAMP somewhere on a cheap / free hosting (e.g. Amazon AWS free tier). I access via ssh and at least know some basic Linux commands .I can adjust php.ini and restart apache, analyze the logs, can see the load (at least see top command), I can create cronjobs and schedule some tasks with them. Regarding the client side, I can do some basic image processing with Photoshop, can pick needed layers and cut graphics. I use Chrome -> right click -> Inspect element and can play with css settings and when I get to the needed values I copy-paste them into my css file.
11. Show me some examples of your work.
I am happy to see:
- infinite scroll in native JS
- stretchable design (not different CSS for different screen resolutions and devices) clear and meaningful names of variable and objects.
- reusable code wrapped in short functions
- each functions no longer that 40 lines of code (to be able to see it all without scrolling)
- validations wherever possible e.g.:
Good:
Bad:
So these questions and 30 minutes of talk help me understand whether a candidate is worth going forward. I am ready to give them a chance to learn or refresh something in their memory. I want to see how they think and work, not what they learned by heart.
I remember my university professor saying "the best practice is theory", which is probably true in some circumstances but for his wisdom we preferred to treat him as our "queer old dean" rather than "dear old queen".
We realise how amazingly someone plays piano when we enjoy the performance, not when we participate in discussions about solfeggio. We have high regards for doctors if they relieve our pains, not if they chew over correct treatment methods.
Multiple choice tests
For example, if I am asked, which function in PHP returns the number of elements in an array: size, sizeof, length, I start hesitating. I simply do not remember. I have been coding in 10 different languages for several different platforms for more than 15 years already and I cannot keep in my memory all syntax in detail. Simply there's no need to do it, because:
- we have code auto completion (hints), so we can always choose from the provided popups.
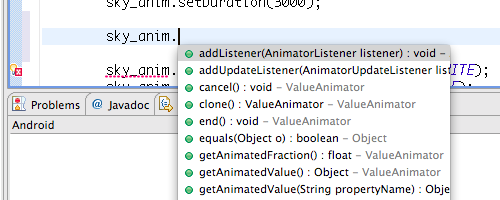
- we, developers, (I am probably telling you now our biggest secret) work in "write once-copy many times" mode. We all have our own sets of libraries, codes, functions which we bring along to each new project and simply copy-paste code snippets.
-we have compilers and error reporters. When a mistake gets into the code (a wrong function or type or number of parameters), the code will not compile and you receive an error with a pointer to exact line where this error sits.
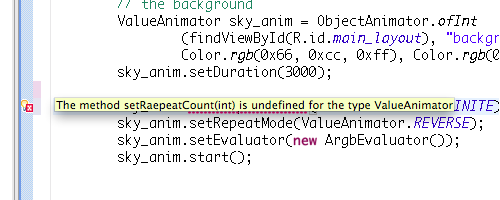
In a multiple choice test I have to choose, I cannot show my work process and cannot explain what I think.
Or another example, you are shown some code and asked to find an error in it or say what it will output. No problem if the code is quite simple but if we have an SQL script with several joins and cases in conditions, it gets really annoying. I have no compiler in my head. When I write an SQL query I do it step by step, build simple blocks, test them on the database, see the errors and fix them. Finally I have a bigger chunck of code, efficient in work but hard for human reading.
So I put there comments. Comments help me remember what this piece of code is about and if I come back to it in 3 years, I will know exactly what it is for and why it was constructed this very and not the opposite way. Or if someone else has to deal with my code, my comments provide the context in a human-friendly format. Have you ever seen multiple choice tests with in-code comments? I have not.
Even worth, tests through at you pieces of code with meaningless methods, variables and classes - no context at all - something like
B = A->foo(c);
If in the real life someone writes such code, he or she deserves been fired immediately.
Development skills should be tested on a real task, in a real working environment: computer + Internet (to be able to check the syntax if needed) + compiler or web server to see immediately how you code works. An alternative is to have a discussion so the tester can see how you react, think, approach problems.
Another good field is object oriented. Don't forget, it has been a holy war arena for ages and the sords are still sharp on both sides. I still remember the times when object-oriented was not so much in fashion (and somehow complex software solutions for space ships worked without object oriented implementations).
Some of my products were written in a procedural approach though the languages allowed object-oriental and they were delivered in time and on budget. Some “theory-savvy” guys laughed at me and my ignorance, but my products are still online while theirs failed initial tests and were rejected. Done is better than perfect.
Of course, candidates have to understand the principles of encapsulation, inheritance and polymorphism, but why on Earth should I test them for these if the project has no hierarchy of objects, everything is quite “flat”?
Now here are my questions for a PHP developer tests:
1. You have a string of values, separated by commas, how to convert it to an array?
I am happy to hear: there're explode and implode functions, I do not remember which is which, but there's php.net and I can check it in 5 secs.
2. Is PHP case sensitive?
I am happy to hear: yes and no. Variables are yes, functions are not, but to be on the safe side, I will code as if everything is case sensitive. The same for any other language. Just for the sake of the discipline.
3. What is an SQL injection and how to prevent it?
I am happy to hear: when through user input some malicious code is sent, it appears in the SQL query and is executed with it. In order to prevent it, we have to filter all user inputs on the server side (even if it's already validated on the client side), and for my personal paranoia, also each incoming parameter to each function must be also validated. And we should check the data types (cast if necessary), check for the reasonable length (and trim), filter out dangerous characters (slashes, quotes, etc). And we have to implement our own logs and log all incoming data and outgoing results (surely we need to have a switch to turn the logs on and off when needed).
4. What happens if 2 simultaneous processes try to update the same database record? And what to do with it?
I am happy to hear: depends on the database and its settings. The second one can wait (good - they both will work, bad - the execution time suffers). Or there might be a dead lock and data losses, uncommitted reads and execution time suffers again. Transactions or isolation levels can help. And all this is good to do in stored procedures.
5. What to do if you work with WordPress and some theme for it and you need to do customization or find a bug in this theme?
I am happy to hear: for customization we need to create a child out of this theme and do the changes in the child. If the original theme is updated, our changes will not suffer.
6. How to post a request from a php script to a remote server and get the result, assume, it's XML and then parse it?
I am happy to hear: there's CURL library, quite powerful, it supports POST and GET methods, caching and sessions and we can even simulate user login to a remote site and then parse the data we receive and in case of XML we have XML parser. I do not remember details, but I am not banned on php.net.
7. Imagine we have 2 web servers with our PHP application and they sit behind a load balancer. A user logins successfully and then in some point in time the user is refused in further actions with “you are not authorised” message. We checked all the system in the test environment, everything is ok. What to do?
I am happy to hear: probably in your test environment there's no load balancer. The problem is that the load balancer can send 1st request to the 1st server and the 2nd to the 2nd, so the 1st request is login and a session is created for this user on the 1st server, but the second request comes to the 2nd server where there's no their session. This happens because by default PHP stores sessions in files. A quick and simple fix is to modify the session management so that it would work with the database, after all the 2 web servers “speak” to the same database.
8. What is AJAX, please show me you implementation?
I am not happy: to see AJAX example based on jQuery and other libraries. It might be good at work, but I want to see native JS code.
I am happy to see something like this:
var xmlhttp; if (window.XMLHttpRequest){ xmlhttp=new XMLHttpRequest(); } else{ xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.onreadystatechange=function(){ if (xmlhttp.readyState==4 && xmlhttp.status==200){ alert (xmlhttp.responseText) } } xmlhttp.open("POST",aURL,true); xmlhttp.setRequestHeader("Content-type", "application/x-www-form-urlencoded"); xmlhttp.send(aParams);
9. If you develop a widget for a web site an you need a specific version of jQuery, but there might be already loaded some other version of jQuery (by other widgets or by the site itself), so conflicts are possible. What to do?
I am happy to hear: wrap my code in its own namespace.
10. Tell me about your work environment.
I am happy to hear: I have a LAMP somewhere on a cheap / free hosting (e.g. Amazon AWS free tier). I access via ssh and at least know some basic Linux commands .I can adjust php.ini and restart apache, analyze the logs, can see the load (at least see top command), I can create cronjobs and schedule some tasks with them. Regarding the client side, I can do some basic image processing with Photoshop, can pick needed layers and cut graphics. I use Chrome -> right click -> Inspect element and can play with css settings and when I get to the needed values I copy-paste them into my css file.
11. Show me some examples of your work.
I am happy to see:
- infinite scroll in native JS
- stretchable design (not different CSS for different screen resolutions and devices) clear and meaningful names of variable and objects.
- reusable code wrapped in short functions
- each functions no longer that 40 lines of code (to be able to see it all without scrolling)
- validations wherever possible e.g.:
Good:
var o = document.getElementById (“div_loading”); if (o){ o.style.display = "block"; o.innerHTML = “Loading”; }
Bad:
var o = document.getElementById (“div_loading”); o.style.display = "block"; o.innerHTML = “Loading”; // this code will fail if there's no div_loading on the page
So these questions and 30 minutes of talk help me understand whether a candidate is worth going forward. I am ready to give them a chance to learn or refresh something in their memory. I want to see how they think and work, not what they learned by heart.
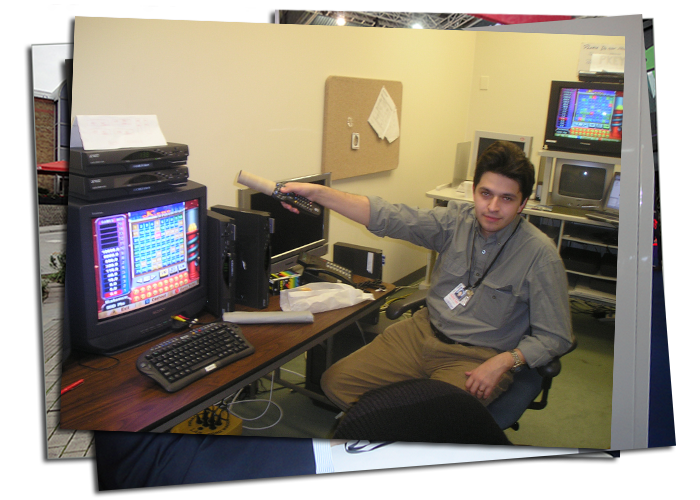
J.Baytelman | 2013 |